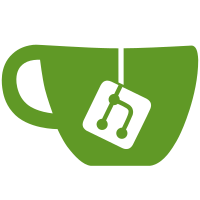
Minor Modifications: * Indentation issues are fixed * Typo errors in source code and comments are fixed * Misspelled parameters in plotTaskFailureReason.m are corrected * sim_results folder is added to .gitignore file Backward compatible changes * The exit code of the application when there is an error is changed from 0 to 1 * Default constructors are added for Location.java, LoadGeneratorModel.java, MobilityModel.java, EdgeOrchestrator.java (this change request coming from a developer) * public TaskProperty(int mobileDeviceId, double startTime, ExponentialDistribution[] expRngList) is added to TaskProperty.java (this change request coming from a developer to create a task without task type) * double getCreationTime() function is added to Task.java * void reconfigureMips(double mips) function is added to EdgeVM (for future usage) * gsm_propagation_delay variable is added to config file. SimSettings class is also modified accordingly. You can use it if you have cellular network access in your scenario. * wlan_range, northern_bound, southern_bound, eastern_bound, western_bound variables are added to config file; and relevant functions are added to SimSettings class. (this change request coming from a developer) Backward incompatible changes! * location_check_interval variable name is changed to location_check_interval in config.properties file. Please update your config files accordingly (remove 'vm_' part) * Major modifications are applied in SimLogger class to decrease time complexity. Now the basic results are kept in the memory and saved to the files at the end of the simulation. As a result of this change, the signature of the SimLogger.addLog () function had to be changed. You must add the mobile device id as the first argument. Please update your MobileDeviceManager class accordingly (add task.getCloudletId () as the first argument).
51 lines
1.4 KiB
Java
51 lines
1.4 KiB
Java
/*
|
|
* Title: EdgeCloudSim - CloudVM
|
|
*
|
|
* Description:
|
|
* CloudVM adds vm type information over CloudSim's VM class
|
|
*
|
|
* Licence: GPL - http://www.gnu.org/copyleft/gpl.html
|
|
* Copyright (c) 2017, Bogazici University, Istanbul, Turkey
|
|
*/
|
|
|
|
package edu.boun.edgecloudsim.cloud_server;
|
|
|
|
import java.util.ArrayList;
|
|
import java.util.List;
|
|
|
|
import org.cloudbus.cloudsim.CloudletScheduler;
|
|
import org.cloudbus.cloudsim.Vm;
|
|
|
|
import edu.boun.edgecloudsim.core.SimSettings;
|
|
|
|
public class CloudVM extends Vm {
|
|
private SimSettings.VM_TYPES type;
|
|
|
|
public CloudVM(int id, int userId, double mips, int numberOfPes, int ram,
|
|
long bw, long size, String vmm, CloudletScheduler cloudletScheduler) {
|
|
super(id, userId, mips, numberOfPes, ram, bw, size, vmm, cloudletScheduler);
|
|
|
|
type = SimSettings.VM_TYPES.CLOUD_VM;
|
|
}
|
|
|
|
public SimSettings.VM_TYPES getVmType(){
|
|
return type;
|
|
}
|
|
|
|
/**
|
|
* dynamically reconfigures the mips value of a VM in CloudSim
|
|
*
|
|
* @param mips new mips value for this VM.
|
|
*/
|
|
public void reconfigureMips(double mips){
|
|
super.setMips(mips);
|
|
super.getHost().getVmScheduler().deallocatePesForVm(this);
|
|
|
|
List<Double> mipsShareAllocated = new ArrayList<Double>();
|
|
for(int i= 0; i<getNumberOfPes(); i++)
|
|
mipsShareAllocated.add(mips);
|
|
|
|
super.getHost().getVmScheduler().allocatePesForVm(this, mipsShareAllocated);
|
|
}
|
|
}
|